Let’s start with Powershell – part1
What’s a Powershell
PowerShell is provided by Microsoft Corporation and is a very usable and powerful scripting language. It’s an object oriented shell.
The standard form of the command in Powershell is:
verb-noun
verb is indicating the action and a noun indicating the object for example:
get-childitem, remove-Item
PowerShell itself is provided as a command line environment. In addition to all the PowerShell “cmdlets” You can run a non-PowerShell program directly (e.g. notepad.exe).
Let’s get started
Powershell is similar to other scripting languages, so knowledge of bash can be helpful. You can use pipes “|”, to pass one object to another command.
To Run powershell:
- Click on the Start button
- Type PowerShell
- You should now see:
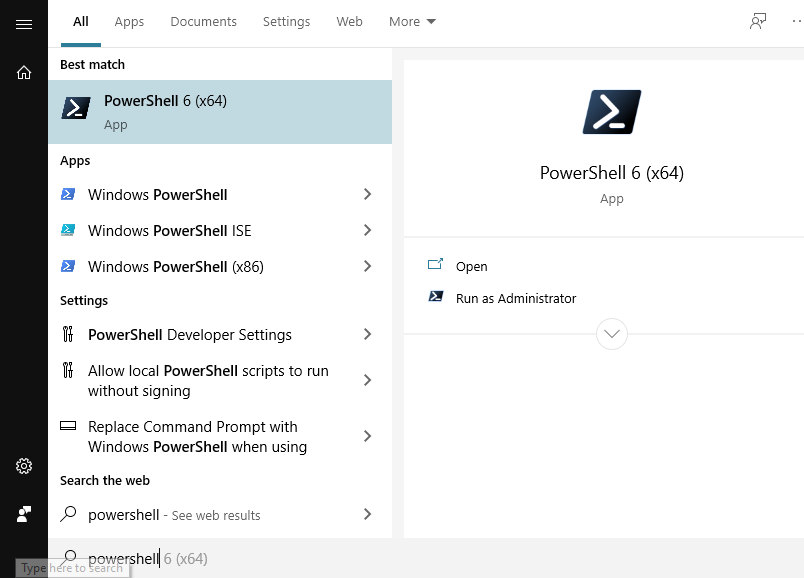
Windows Powershell is a like CMD window. Ideal to run simple command, or run scripts
Windows Powershell ISE is a tool to write scripts. On this course, we will use Powershell ISE
Windows Powershell 6 is a new version of the powershell. It’s not installed in the system by default.
Commands
There are few command that will be helpful during this course:
Get-command – This is a way to find a command that we are looking for, but don’t know the exact name
Get-command *file – will give us a full list of commands with the word file at the end.
PS C:\> get-command *file CommandType Name Version Source ----------- ---- ------- ------ Alias Set-AppPackageProvisionedDataFile 3.0 Dism Alias Set-ProvisionedAppPackageDataFile 3.0 Dism Alias Set-ProvisionedAppXDataFile 3.0 Dism Function Close-SmbOpenFile 2.0.0.0 SmbShare Function Disable-NetIPHttpsProfile 1.0.0.0 NetworkTransition Function Enable-NetIPHttpsProfile 1.0.0.0 NetworkTransition Function Get-NetConnectionProfile 1.0.0.0 NetConnection Function Get-NetFirewallProfile 2.0.0.0 NetSecurity Function Get-SmbOpenFile 2.0.0.0 SmbShare Function Import-PowerShellDataFile 3.1.0.0 Microsoft.PowerShell.Utility Function New-TemporaryFile 3.1.0.0 Microsoft.PowerShell.Utility Function Set-NetConnectionProfile 1.0.0.0 NetConnection Function Set-NetFirewallProfile 2.0.0.0 NetSecurity Function Set-StorageBusProfile 1.0.0.0 StorageBusCache Cmdlet Add-BitsFile 2.0.0.0 BitsTransfer Cmdlet New-PSRoleCapabilityFile 3.0.0.0 Microsoft.PowerShell.Core Cmdlet New-PSSessionConfigurationFile 3.0.0.0 Microsoft.PowerShell.Core Cmdlet Out-File 3.1.0.0 Microsoft.PowerShell.Utility Cmdlet Set-AppXProvisionedDataFile 3.0 Dism Cmdlet Set-UevTemplateProfile 2.1.639.0 UEV Cmdlet Test-PSSessionConfigurationFile 3.0.0.0 Microsoft.PowerShell.Core Cmdlet Unblock-File 3.1.0.0 Microsoft.PowerShell.Utility
You can use wildcarts also at the end. Get-command *file* will return all the commands with “file” word.
Get-help – This command will list the help for the desired command
Get-help Out-File
PS C:\> help Out-File NAME Out-File SYNTAX Out-File [-FilePath] <string> [[-Encoding] {unknown | string | unicode | bigendianunicode | utf8 | utf7 | utf32 | ascii | default | oem}] [-Append] [-Force] [-NoClobber] [-Width <int>] [-NoNewline] [-InputObject <psobject>] [-WhatIf] [-Confirm] [<CommonParameters>] Out-File [[-Encoding] {unknown | string | unicode | bigendianunicode | utf8 | utf7 | utf32 | ascii | default | oem}] -LiteralPath <string> [-Append] [-Force] [-NoClobber] [-Width <int>] [-NoNewline] [-InputObject <psobject>] [-WhatIf] [-Confirm] [<CommonParameters>] ALIASES None REMARKS Get-Help cannot find the Help files for this cmdlet on this computer. It is displaying only partial help. -- To download and install Help files for the module that includes this cmdlet, use Update-Help. -- To view the Help topic for this cmdlet online, type: "Get-Help Out-File -Online" or go to https://go.microsoft.com/fwlink/?LinkID=113363.
Write-host – will write to the screen. It can be used with parameters. If no parameter is used it will create a blank line of output.
With write-host You can specify the color of text by using the ForegroundColor parameter , and you can specify the background color by using the BackgroundColor parameter . The Separator parameter lets you specify a string to use to separate displayed objects.
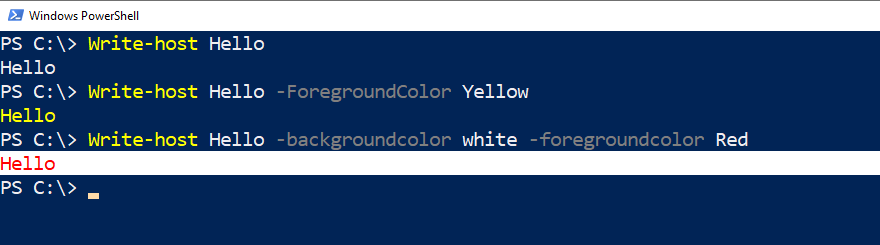
There is a similar command write-output. This is used, when You want to send it through the pipeline, but not necessarily to display.
PS H:\> write-output ala >a.txt PS H:\> type .\a.txt ala PS H:\> write-host ala >a.txt ala PS H:\> type .\a.txt PS H:\>
In the example Write-host wrote the “ala” on the screen but didn’t wrote in in file.
Write-output wrote it in file, but didn’t wrote on the screen
Command Aliases
In the powershell we can use a lot of aliases for the commands. For those who have used DOS or Unix these can be very familiar. Aliases are short forms of a command. So for someone used to using the command pwd typing help pwd will indicate that the underlying command is actually get-location . However, if coming from a Unix or DOS environment, typing the form you are familiar with makes adopting powershell easier.
clear-host cls, clear format-list fl get-childitem gci, ls, dir get-content gc, cat, type get-location gl, pwd get-member g m remove-item ri, rm, rmdir, del, erase, rd write-output write, echo
Exercise
- Type write-host “Hello World” and press the Enter key
- Type write-host -foregroundcolor yellow “Hello World”
- Get PowerShell to print in blue text on a yellow background?
- Type help clear-host